In this post, I’ll show you how to embed Google map on website and generate a map with your own icons.
Creating API keys:
To create an API key follow these steps:
- Go to the APIs & Services > Credentials page.
- On the Credentials page, click Create credentials > API key. The API key created dialog displays your newly created API key.
- The new API key is listed on the Credentials page under API keys.

Generating a map:
You must include an API key in your project request. Replace YOUR_API_KEY
with your API key.
https://maps.googleapis.com/maps/api/js?key=YOUR_API_KEY&callback=initMap
Create a index.html file and insert below code.
<!DOCTYPE html>
<html>
<head>
<title>Simple Markers</title>
<script src="https://polyfill.io/v3/polyfill.min.js?features=default"></script>
<script src="https://maps.googleapis.com/maps/api/js?key=YOUR_API_KEY&callback=initMap" defer></script>
</head>
<body>
<div id="map"></div>
</body>
</html>
Adding Markers Label:
function initMap() {
const myLatLng = { lat: -24, lng: 130 };
const map = new google.maps.Map(document.getElementById("map"), {
zoom: 4,
center: myLatLng,
});
new google.maps.Marker({
position: myLatLng,
map,
title: "The Knowledge Adda",
});
}
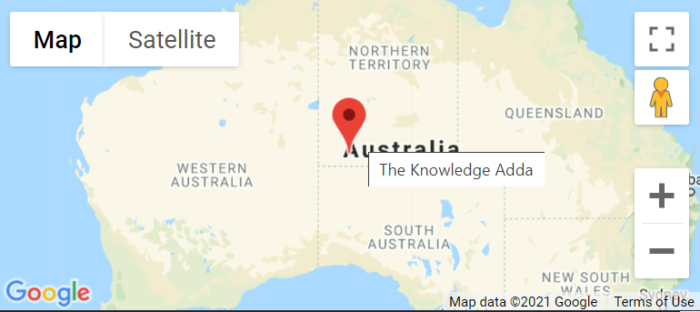
Adding Markers Icon:
function initMap() {
const map = new google.maps.Map(document.getElementById("map"), {
zoom: 4,
center: { lat: -24, lng: 130 },
});
const image =
"https://developers.google.com/maps/documentation/javascript/examples/full/images/beachflag.png";
const beachMarker = new google.maps.Marker({
position: { lat: -26.89, lng: 133.274 },
map,
icon: image,
});
}
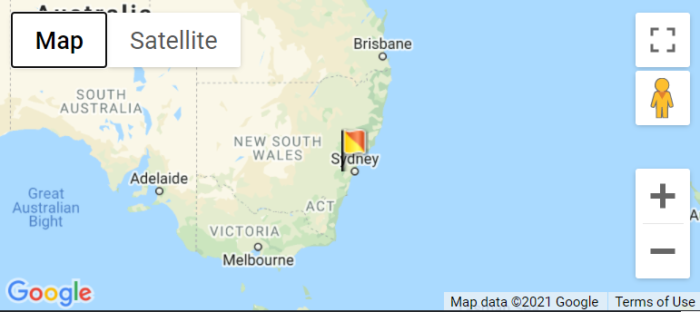
Thanks for sharing your thoughts. I truly appreciate your
efforts and I am waiting for your next write ups thank you once again.
Great article! We are linking to this particularly great content on our website.
Keep up the good writing.
Pretty nice post. I just stumbled upon your blog and wished to
say that I have truly enjoyed browsing your blog posts.
In any case I will be subscribing to your rss feed and
I hope you write again very soon!
Heya i’m for the first time here. I found this board and I find It really useful &
it helped me out much. I hope to give something back and aid others like you aided me.
Very good info. Lucky me I came across your website
by accident (stumbleupon). I’ve book-marked it for later!
Excellent post. I was checking continuously this blog and
I am impressed! Extremely useful information specially the
last part 🙂 I care for such info a lot. I was seeking
this certain information for a very long time. Thank you and best of luck.
Hi, i think that i saw you visited my weblog thus i came to “return the favor”.I am attempting to
find things to enhance my web site!I suppose its ok to use a few of your ideas!!
Check out my website … delta 8 gummies (http://www.seattleweekly.com)
This paragraph will assist the internet viewers for building
up new weblog or even a blog from start to end.
Thank you a lot for sharing this with all people you actually realize what you are talking approximately!
I loved as much as you’ll receive carried out right here.
The sketch is attractive, your authored subject matter stylish.
nonetheless, you command get bought an edginess over that you wish
be delivering the following. unwell unquestionably come more formerly again since exactly the
same nearly very often inside case you shield this hike.
First of all I want to say great blog! I had a quick question that I’d like to
ask if you do not mind. I was interested to find
out how you center yourself and clear your mind prior to writing.
I have had a hard time clearing my thoughts in getting my
thoughts out there. I truly do take pleasure in writing however it just seems like the
first 10 to 15 minutes are generally lost simply just trying
to figure out how to begin. Any suggestions or hints? Kudos!
Valuable information. Fortunate me I found your website by accident,
and I am surprised why this twist of fate did not took place earlier!
I bookmarked it.
Dart is a programming language that is used to develop mobile and desktop applications. We develop a tool that converts JSON into a dart class with null safety. JSON to dart converter tool is easy to use. Just copy and paste your JSON into the textbox and hit the dart converter button. JSON to dart null safety feature is default enabled into the script.
Json Beautifier Online tool
I don’t even know how I finished up here, but I thought this put up used to be great. I don’t know who you are but certainly you are going to a well-known blogger when you are not already 😉 Cheers!
Great article, exactly what I was looking for.
Good web site! I truly love how it is simple on my eyes and the data are well written. I’m wondering how I could be notified when a new post has been made. I’ve subscribed to your RSS feed which must do the trick! Have a great day!
Good – I should definitely pronounce, impressed with your site. I had no trouble navigating through all the tabs and related information ended up being truly simple to do to access. I recently found what I hoped for before you know it at all. Quite unusual. Is likely to appreciate it for those who add forums or something, site theme . a tones way for your customer to communicate. Excellent task.